DBOS Transact is an ultra-lightweight open source durable execution library (Python and TypeScript) with built-in once-and-only-once code execution. You simply write your business logic and DBOS Transact makes sure it executes correctly–you no longer need to worry about manually coding retries and recovery procedures.
In this post, we’ll introduce you to the capabilities of the DBOS Transact library, the problems it solves, and how it relates to DBOS Cloud.
Solving the transactional execution problem
The most exciting feature of DBOS Transact is transactional execution. Any program written with DBOS Transact is guaranteed to run to completion and each of its operations is guaranteed to execute once and only once, with complete auditability. As such, DBOS Transact is a good fit for use cases like:
- Payment processing
- Workflows - synchronous or asynchronous, short- or long-running
- Applications managing sensitive data
- Data preparation/transformation pipelines
- Supply chain and inventory logistics
For example, let’s say you want to write an application that sells tickets to a concert. Its flow might look like this:
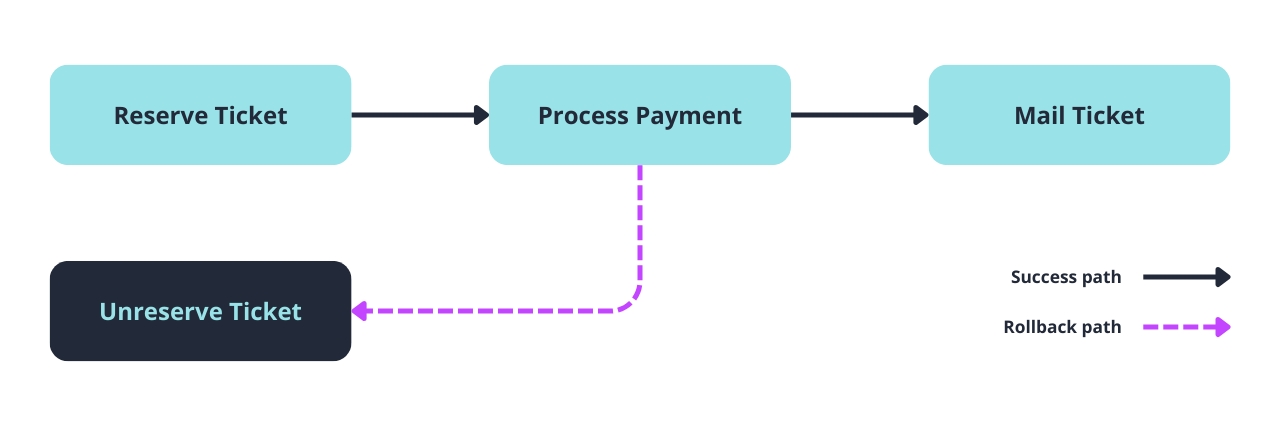
This application is tricky because you have to account for failures during any step. For example, if your application is interrupted after a customer pays but before you send them their tickets, you have to make sure it recovers and sends them their tickets without charging them again. If you’re not careful, your customers will, at best, get frustrating errors like this (and at worst, see a ticket they paid for never arrive):

In most frameworks, making your application reliable requires a lot of complex code. For example, you might have to code up your entire application as a state machine, manually persist your state to the database, and write a helper method that can recover from any state. By contrast, DBOS Transact automatically recovers, so you can focus on building your application instead of worrying about handling failures.
Under the hood, DBOS Transact works by automatically recording key steps your application takes in the database. If steps perform database operations, it records them in the same transactions as their business logic. This way, even if your application is interrupted, DBOS Transact can look up your unfinished programs in the database and resume them from where they left off.
Decorating your Python and TypeScript code with DBOS Transact
Using DBOS Transact is easy. You write your business logic in ordinary Python and TypeScript functions, then annotate them with “decorators” to obtain powerful features, such as durable workflow execution, automatic observability (OpenTelemetry traces), access control, and more. For example, in this code snippet we implement the ticketing workflow from the above example.
Here, the @Workflow decorator directs DBOS Transact to run the checkoutTicket function as a reliable workflow, guaranteeing each step executes once and only once.
Run it anywhere
Applications built with the DBOS Transact library can run anywhere: on your laptop or in your cloud. All you need is a PostgreSQL-compatible DBMS to store your application’s state and data. At DBOS, we also provide a hosting solution optimized for DBOS Transact: DBOS Cloud. More on that below!
Testing and deploying DBOS Transact applications
Because it runs anywhere (including your laptop!) DBOS Transact is often used for local development and testing of transactional application backends. However, if you plan to deploy DBOS Transact applications into production, we recommend you use DBOS Cloud. This gives you a number of benefits:
- Push-button, serverless application deployment
- Automatic application scaling as your demand evolves.
- Automatic application recovery, leveraging transactional execution
- Automatic collection of metrics, logs, and traces with dashboard visualization
- Time-travel debugging - view application execution at any point in time, stepping through state changes as they actually happened
DBOS Cloud has a free tier you can use to evaluate the full power of DBOS Transact. Try it today!
Give it a try - we’d love your feedback
To get started building applications with DBOS Transact, check out the quickstart.
To learn about deploying applications to DBOS Cloud, check out our quickstart and application examples.
You can discuss DBOS Transact with us or with other users via the DBOS community on Discord. We look forward to chatting with you!